How to draw a pie slice - Attempts included
Posted: 2009-04-11T08:16:51-07:00
Been pulling my hair out over how to draw a pie slice, nothing I do seems to work.
If someone could tell me how to do it, it would be greatly appreciated.
Code below is my testcase, bottom left image is close.. and although the borders seem somewhat 'arced' it isn't exactly nice a circle.. more like the design of stealth bomber with those triangular edges.
If someone could tell me how to do it, it would be greatly appreciated.
Code below is my testcase, bottom left image is close.. and although the borders seem somewhat 'arced' it isn't exactly nice a circle.. more like the design of stealth bomber with those triangular edges.
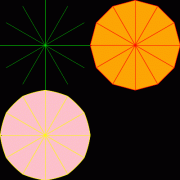
Code: Select all
<?php
function getPointOnCircumference( $widthOfCircle, $heightOfCircle, $degrees, $x = 0, $y = 0 )
{
return array(
'x' => $x + ($widthOfCircle/2) * sin( deg2rad( $degrees ) ),
'y' => $y + ($heightOfCircle/2) * cos( deg2rad( $degrees ) )
);
}
$width = 400;
$height = 400;
$x = $width / 2;
$y = $height / 2;
$im = new Imagick();
$im->newImage( $width, $height, "black", "png" );
$draw1 = new ImagickDraw();
$draw1->setFillColor( 'lime' );
$draw1->pathStart();
for( $i = 0; $i < 360; $i+=30 )
{
$draw1->pathMoveToAbsolute( $x/2, $y/2 ); //Move 'pencil' to middle of image.
$point = getPointOnCircumference( $width/2, $height/2, $i );
$draw1->pathLineToRelative( $point['x'], $point['y'] );
}
$draw1->pathClose();
$im->DrawImage( $draw1 );
$draw2 = new ImagickDraw();
$draw2->setFillColor( 'orange' );
$draw2->setStrokeColor( 'red' );
$draw2->pathStart();
for( $i = 0; $i < 360; $i+=30 )
{
$draw2->pathMoveToAbsolute( $x+$x/2, $y/2 ); //Move 'pencil' to middle of image.
$point = getPointOnCircumference( $width/2, $height/2, $i );
$draw2->pathLineToRelative( $point['x'], $point['y'] );
$point = getPointOnCircumference( $width/2, $height/2, $i+30 );
$draw2->pathLineToAbsolute( ($x+$x/2)+$point['x'], $y/2+$point['y'] );
}
$draw2->pathClose();
$im->DrawImage( $draw2 );
$draw3 = new ImagickDraw();
$draw3->setFillColor( 'pink' );
$draw3->setStrokeColor( 'yellow' );
$draw3->pathStart();
for( $i = 0; $i < 360; $i+=30 )
{
$draw3->pathMoveToAbsolute( $x/2, $y+$y/2 ); //Move 'pencil' to middle of image.
$point = getPointOnCircumference( $width/2, $height/2, $i );
$draw3->pathLineToRelative( $point['x'], $point['y'] );
$point = getPointOnCircumference( $width/2, $height/2, $i+30 );
$draw3->pathEllipticArcAbsolute( $width/2, $height/2, 0, false, false, $x/2+$point['x'], ($y+$y/2)+$point['y'] );
}
$draw3->pathClose();
$im->DrawImage( $draw3 );
header( "Content-Type: image/png" );
echo $im;
?>